Updated on May 24, 2018 with code based from Essence Pro.
Certain Genesis child themes from StudioPress like Essence Pro have the Flexible Widgets feature wherein the widgets placed in widget areas automatically get arranged in different layouts on the frontend depending on the number of widgets in the widget area.
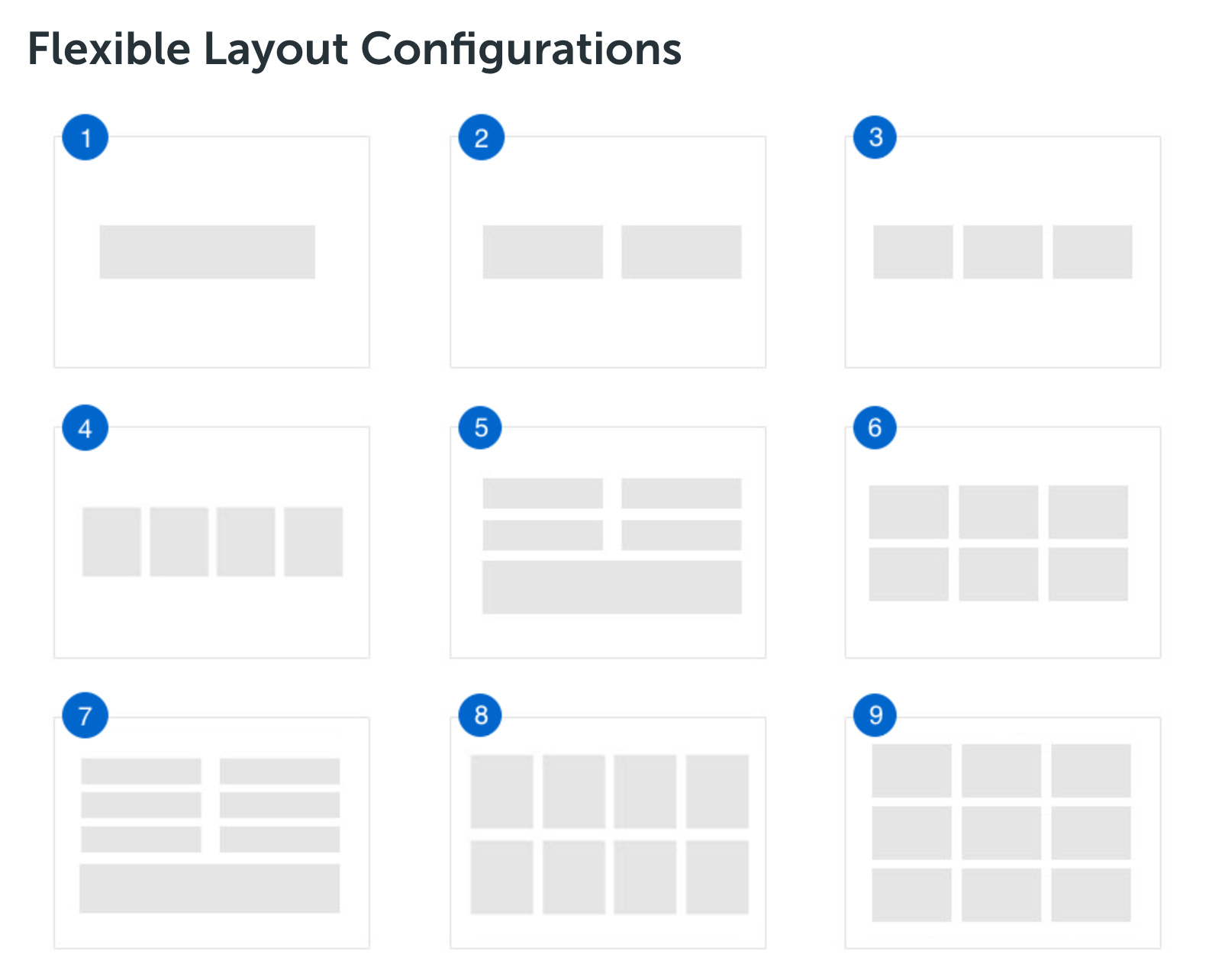
In this article, I share code taken from Essence Pro for flexible layout widgets with a few tweaks that can be used in any Genesis child theme.
Step 1
Add the following in child theme’s functions.php:
/**
* Counts used widgets in given sidebar.
*
* @param string $id The sidebar ID.
* @return int|void The number of widgets, or nothing.
*/
function flexible_count_widgets( $id ) {
$sidebars_widgets = wp_get_sidebars_widgets();
if ( isset( $sidebars_widgets[ $id ] ) ) {
return count( $sidebars_widgets[ $id ] );
}
}
/**
* Gives class name based on widget count.
*
* @param string $id The widget ID.
* @return string The class.
*/
function flexible_widget_area_class( $id ) {
$count = flexible_count_widgets( $id );
$class = '';
if ( 1 === $count ) {
$class .= ' widget-full';
} elseif ( 0 === $count % 3 ) {
$class .= ' widget-thirds';
} elseif ( 0 === $count % 4 ) {
$class .= ' widget-fourths';
} elseif ( 1 === $count % 2 ) {
$class .= ' widget-halves uneven';
} else {
$class .= ' widget-halves';
}
return $class;
}
/**
* Helper function to handle outputting widget markup and classes.
*
* @param string $id The id of the widget area.
*/
function flexible_do_widget( $id ) {
$count = flexible_count_widgets( $id );
$columns = flexible_widget_area_class( $id );
genesis_widget_area(
$id, array(
'before' => '<div id="' . $id . '" class="' . $id . '"><div class="flexible-widgets widget-area ' . $columns . '"><div class="wrap">',
'after' => '</div></div></div>',
)
);
}
Step 2
Add the following in child theme’s style.css:
/* Flexible Widgets
--------------------------------------------- */
.flexible-widgets .widget {
float: left;
margin-bottom: 0;
padding: 40px;
background-color: #fff;
}
.flexible-widgets .widget table {
margin-bottom: 0;
}
.flexible-widgets.widget-full .widget,
.flexible-widgets.widget-halves.uneven .widget:last-of-type {
width: 100%;
}
.flexible-widgets.widget-fourths .widget {
width: 22%;
margin-left: 4%;
}
.flexible-widgets.widget-halves .widget {
width: 48%;
margin-left: 4%;
}
.flexible-widgets.widget-thirds .widget {
width: 31%;
margin-left: 3.5%;
}
.flexible-widgets.widget-halves .widget:nth-of-type(odd),
.flexible-widgets.widget-thirds .widget:nth-child(3n + 1),
.flexible-widgets.widget-fourths .widget:nth-child(4n + 1) {
clear: left;
margin-left: 0;
}
.flexible-widgets .featured-content {
padding: 0;
background-color: transparent;
-webkit-box-shadow: none;
box-shadow: none;
}
.flexible-widgets .featured-content .entry {
background-color: #fff;
-webkit-box-shadow: 0 25px 60px 0 rgba(0, 0, 0, 0.05);
box-shadow: 0 25px 60px 0 rgba(0, 0, 0, 0.05);
text-align: center;
}
.flexible-widgets .featured-content .entry-title {
font-size: 26px;
font-size: 2.6rem;
text-decoration: none;
letter-spacing: -1px;
}
.flexible-widgets .featured-content .entry-content,
.flexible-widgets .featured-content .entry-title {
padding: 15px 40px 0 40px;
}
.flexible-widgets .featuredpost .entry-meta {
padding-right: 40px;
padding-left: 40px;
}
.flexible-widgets.widget-full .featuredpost .entry,
.flexible-widgets.widget-halves.uneven .featuredpost.widget:last-of-type .entry {
float: left;
width: 31%;
margin-left: 3.5%;
}
.flexible-widgets.widget-full .featuredpost .entry:nth-of-type(3n + 1),
.flexible-widgets.widget-halves.uneven .featuredpost.widget:last-of-type .entry:nth-of-type(3n + 1) {
clear: left;
margin-left: 0;
}
.flexible-widgets .featured-content .more-posts-title {
padding: 30px 0;
}
.flexible-widgets .featured-content .more-posts {
text-align: center;
}
.flexible-widgets.widget-full .featured-content .more-posts,
.flexible-widgets.widget-halves.uneven .featured-content:last-of-type .more-posts {
clear: both;
-webkit-column-count: 3;
column-count: 3;
padding-bottom: 30px;
}
.flexible-widgets .featured-content .more-from-category {
margin-bottom: 55px;
}
@media only screen and (max-width: 860px) {
.flexible-widgets .wrap {
padding-left: 0;
padding-right: 0;
}
.flexible-widgets.widget-fourths .widget,
.flexible-widgets.widget-halves .widget,
.flexible-widgets.widget-thirds .widget {
float: none;
margin-left: 0;
width: 100%;
}
.flexible-widgets.widget-full .widget .entry,
.flexible-widgets.widget-halves.uneven .widget:last-of-type .entry {
margin-left: 0;
width: 100%;
}
.flexible-widgets .entry:last-of-type {
margin-bottom: 40px;
}
.flexible-widgets .featured-content .entry-title,
.half-width-entries .flexible-widgets .featured-content .entry-title,
.flexible-widgets.widget-full .entry-title,
.flexible-widgets.widget-halves.uneven .widget:last-of-type .entry-title {
font-size: 28px;
font-size: 2.8rem;
}
.flexible-widgets.widget-full .featured-content .more-posts,
.flexible-widgets.widget-halves.uneven .featured-content:last-of-type .more-posts {
-webkit-column-count: 1;
-moz-column-count: 1;
column-count: 1;
}
}
Step 3
If you haven’t already, register your widget area(s).
Ex.: In functions.php add
// Registers `before-footer` widget area.
genesis_register_widget_area(
array(
'id' => 'before-footer',
'name' => __( 'Before Footer', 'my-theme-text-domain' ),
'description' => __( 'This is the before footer section.', 'my-theme-text-domain' ),
)
);
Step 4
Continuing with the above example, here’s how we set up the custom column class to automatically be applied to the Before Footer widget area based on the number of widgets in it AND output the widget area:
add_action( 'genesis_before_footer', 'sk_before_footer', 7 );
/**
* Adds `before-footer` flexible featured widget area above the footer widgets.
*/
function sk_before_footer() {
flexible_do_widget( 'before-footer' );
}
The important part is flexible_do_widget( 'before-footer' );
.
We just need to pass in the ID of widget area that should be shown with the flexible widets magic.
Step 5
Go to Appearance > Widgets and populate your widget areas that have been made flexible with 1 or more widgets.
Reference:
Excellent tutorial, thank you
Sridhar – thank you. Can I use this to convert the Altitude Pro footer widget into a flexible widget area? What adjustments would I make to do that? Thanks.
Follow https://sridharkatakam.com/replace-footer-widgets-altitude-pro-flexible-widgets/
I would like to apply this to an existing widget area, front-page-4, I am not quite sure how to adapt this. Thanks appreciate it!!
Which child theme are you using?
Monochrome Pro
Monochrome Pro includes the code for Flexible widgets and the Front Page 4 (and others) are flexible out of the box. There’s no need to implement this tutorial in Monochrome Pro.
http://marketleadshop.com/ frontpage-4 has two widgets in it but they are each 100%- bottom of home page
https://www.screencast.com/t/UCCFQq7Lc
aah, this is one of those SP themes where the flexible widget layout is different/old (https://s3.amazonaws.com/screensteps_live/image_assets/assets/000/534/521/original/91ba0490-86a2-415e-8794-000c70200356.jpg?1494335984).
Try this:
1) In Step 2, replace all instances of
.flexible-widgets
with.custom-flexible-widgets
.2) In front-page.php, replace
with
I tried to add the last code in step4 but it returns error…
Uncaught Error: Call to undefined function custom_widget_area_class() in wp-content/themes/Wesharethis vs2/functions.php:960
Stack trace:
#0 wp-includes/class-wp-hook.php(286): sk_home_featured(”)
#1 wp-includes/class-wp-hook.php(310): WP_Hook->apply_filters(NULL, Array)
#2 wp-includes/plugin.php(453): WP_Hook->do_action(Array)
#3 wp-content/themes/genesis/header.php(35): do_action(‘genesis_after_h…’)
#4 wp-includes/template.php(688): require_once(‘/home/wesheyyl/…’)
#5 wp-includes/template.php(647): load_template(‘/home/wesheyyl/…’, true)
#6 wp-includes/general-template.php(41): locate_template(Array, true)
#7 wp-content/themes/genesis/lib/framework.php(24): get_header()
#8 wp-content/themes/Wesharethis vs2/home.php(5): genesis()
#9 /ho
Did you do Step 1?
Yes I do… I followed the guidelines accordingly..
Which Genesis child theme are you using?
Am using genesis example child theme
I’d need to log into your site’s admin to troubleshoot.
If you are paid subscriber/member of this site, you can contact me via https://sridharkatakam.com/contact regarding this.
Actually am a regular reader of this blog but havent subscribed to your membership yet…I think you can still assist in another way in this situation… I will consider the subscription for other time..
Warm regards
hi sridar,
I tried it in your starter team and I found that the .flexible-widgets .wrap requires overflow: hidden to avoid content of one front-page wrap to be displayed on the following. I am not a dev, but I added a background to the front-page classes and I discovered such a “bug”. hope it helps, in my experience this code solved the problem
.flexible-widgets .wrap {
max-width: 1280px;
padding: 80px 0 40px;
overflow: hidden; /* added by me */
}
I am trying to use this Enterprise-Pro and the widgets just stack in the left 1/3 of flexible widget area. Might you have any advice to make this work as it should?
Replied to your email.
Is it possible to apply this to all the front page sections of the SP parallax theme?
It does not seem to work with latest Studipress Genesis Sample Theme.
The widgets are not displayed in “Appearance > Widgets”. Though there is a message there about the 5 widgets. See screenshot at: http://prntscr.com/khh52y
Can you change the language to English and share the screenshot again?
I just say there is 5 widget areas but they are not shown…
Hi,
Can I use this code to adda completely new flexible widget area to Essence Pro?
Thanks!
Chip
Hi Chip,
To add a new flexible widget area in Essence Pro, you just need to add code similar to
wherever you want the widget area to be output.
Replace
before-footer
with the id of your widget area.Hi Sridhar,
I am using Enterprise Pro and wish to use this tutorial for the Home – Bottom widget area. I see you replied to someone else above regarding Enterprise Pro. Is there anything different I should do?
Thanks.
Chip
Sridhar – do you have a tutorial for updating an existing flexible widget layout? I’m looking to have a full width widget at the top of a 5 widget & 7 widget layout option (rather than having the full width widget at the bottom. Thanks!
Which theme are you using?
I cannot seem to get this to work, with or without front-page.php. The widget areas are there and populated, but they do not appear on the home page. I’d love some paid help with this project if you have the time. Thanks!!
Site is here: tvhoa.mytestbed.com
I’m using the Genesis Sample theme… Is there a way to move the Flexible Widgets on the Appearance -> Widgets page to the second column?
That is not possible as far as I know w/o using some custom JS hack.
You can try asking this in wp.org forum.