In the last 2 years of working with WordPress, I must have created or customized at least a couple hundred websites using iThemes Builder.
I store all the reusable snippets of iThemes Builder, WordPress and CSS related code in nvALT and want to share them all in this article.
Note that PHP can be enabled in HTML module in Builder.
bloginfo
<?php bloginfo('url'); ?>
= Site homepage URL
<?php bloginfo('name'); ?>
= Site name
<?php bloginfo('description'); ?>
= Site tagline
<?php bloginfo( 'stylesheet_directory' ); ?>/images
= Active child theme directory.
Site title linking to homepage and tagline
<h1 id="site-title"><a href="<?php bloginfo('url'); ?>"><?php bloginfo('name'); ?></a></h1>
<div id="site-tagline"><?php bloginfo('description'); ?></div>
Logo at left and nav at right
In PHP enabled HTML module:
<a id="logo" href="<?php bloginfo('url'); ?>"><img src="<?php bloginfo( 'stylesheet_directory' ); ?>/images/logo.png" alt="Home" /></a>
<?php wp_nav_menu( array( 'menu' => 'top menu', 'menu_class' => 'builder-module-navigation') ); ?>
In style.css:
.menu-top-menu-container {
float: right;
margin-top: 1.8em;
}
Logo linking to site URL
<a id="logo" href="<?php bloginfo('url'); ?>"><img src="<?php bloginfo( 'stylesheet_directory' ); ?>/images/logo.png" alt="Home" /></a>
To add sidebar support for Navigation module
add_theme_support( 'builder-navigation-module-sidebars' );
(goes in functions.php)
Show custom menu in PHP enabled HTML module
<?php wp_nav_menu( array( 'menu' => 'Main Menu', 'menu_class' => 'builder-module-navigation') ); ?>
Display search
<?php get_search_form(); ?>
This outputs contents of searchform.php.
Breadcrumbs
With Yoast’s WordPress SEO plugin active and breadcrumbs enabled at SEO > Internal links,
add_action( 'builder_module_render_element_block_contents_content', 'show_breadcrumbs', 0 );
function show_breadcrumbs() { ?>
<?php if ( function_exists('yoast_breadcrumb') ) {
yoast_breadcrumb('<p id="breadcrumbs">','</p>');
} ?>
<?php }
(goes in functions.php)
Apply a layout using code
function custom_filter_category_layouts( $layout_id ) {
if ( is_single() && ( in_category( 'news' ) || in_category( 'photos' ) ) )
return '4e5f997043d8e';
return $layout_id;
}
add_filter( 'builder_filter_current_layout', 'custom_filter_category_layouts' );
The logic of the if statements equates to “only do the following if the page is a single (a blog post) and the blog post is in either the news or photos category.” Note that the && means “and” while the || means “or.”
(goes in functions.php)
Another example:
function custom_filter_single_project_layout( $layout_id ) {
if ( is_singular('projects') )
return '516b7e8257cdf';
return $layout_id;
}
add_filter( 'builder_filter_current_layout', 'custom_filter_single_project_layout' );
More examples here.
To force element to appear first and then the sidebar below in mobile view
add_theme_support( 'builder-module-column-source-order', 'element-first' );
(goes in functions.php)
To display value of a custom field if not empty
<?php
global $post;
$price = get_post_meta($post->ID, 'price', true);
if ( !empty($price) ) { echo 'Price: ' . $price; }
?>
custom.css
function my_custom_styles() {
wp_enqueue_style( 'custom-style', get_stylesheet_directory_uri() . '/custom.css', '1.0.0', 'all' );
}
add_action('wp_enqueue_scripts', 'my_custom_styles');
(goes in functions.php)
Manual placement of Digg Digg social sharing buttons
<div class="my-social-icons">
<div class="social-pinterest dd-social"><?php if(function_exists('dd_pinterest_generate')){dd_pinterest_generate('Compact');} ?></div>
<div class="social-twitter dd-social"><?php if(function_exists('dd_twitter_generate')){dd_twitter_generate('Compact','twitter_username');} ?></div>
<div class="social-facebook dd-social"><?php if(function_exists('dd_fblike_generate')){dd_fblike_generate('Like Button Count');} ?></div>
<div class="social-google dd-social"><?php if(function_exists('dd_google1_generate')){dd_google1_generate('Compact (20px)');} ?></div>
</div>
and
.my-social-icons {
margin-top: 1em;
margin-bottom: 3em;
}
.dd-social {
float: left;
margin-right: 1em;
}
.social-twitter,
.social-facebook {
width: 75px;
}
Disable WordPress visual editor
add_filter( 'user_can_richedit', 'disable_my_richedit' );
function disable_my_richedit( $default ) {
global $post;
if ( $post->ID == 32 ) // change the post ID
return false;
return $default;
}
where 32 is the ID of Page/Post.
For multiple entries,
add_filter( 'user_can_richedit', 'disable_my_richedit' );
function disable_my_richedit( $default ) {
global $post;
if ( in_array( $post->ID, array(32,33) ) ) // change the post ID
return false;
return $default;
}
(goes in functions.php)
To display shortcodes in PHP template files
<?php echo do_shortcode("[your_shortcode]"); ?>
Easing for hyperlinks and images
a { color: #008bbf; text-decoration:none; outline: 0;
-webkit-transition-property: background color; -webkit-transition-duration: 0.12s; -webkit-transition-timing-function: ease-out;
-moz-transition-property: background color; -moz-transition-duration: 0.12s; -moz-transition-timing-function: ease-out;
-o-transition-property: background color; -o-transition-duration: 0.12s; -o-transition-timing-function: ease-out;
transition-property: background color; transition-duration: 0.12s; transition-timing-function: ease-out; }
a:hover { color: #333; }
a img { -webkit-transition-property: opacity; -webkit-transition-duration: 0.12s; -webkit-transition-timing-function: ease-out;
-moz-transition-property: opacity; -moz-transition-duration: 0.12s; -moz-transition-timing-function: ease-out;
-o-transition-property: opacity; -o-transition-duration: 0.12s; -o-transition-timing-function: ease-out;
transition-property: opacity; transition-duration: 0.12s; transition-timing-function: ease-out; }
a img:hover {opacity:0.7;}
Simpler version:
-webkit-transition: all .2s linear;
-moz-transition: all .2s linear;
-o-transition: all .2s linear;
transition: all .2s linear;
To load Javascript before closing body tag using WordPress enqueue
add_action( 'wp_enqueue_scripts', 'load_my_javascript_files' );
function load_my_javascript_files() {
wp_register_script( 'my-imagemap', get_stylesheet_directory_uri() . '/js/imagemap.js', array('jquery'), '1.0', true );
if ( is_front_page() ) {
wp_enqueue_script('my-imagemap');
}
}
Example 2:
// =========================================
// = My Changes Below =
// =========================================
// load javascript and styles in footer or header
// only on front page
add_action('wp_enqueue_scripts', 'jcarousel_code');
function jcarousel_code() {
if(is_front_page()) {
wp_enqueue_script( 'jcarousel', get_bloginfo('wpurl') . '/assets/jcarousel/lib/jquery.jcarousel.min.js', array('jquery'), '1.0', true );
wp_enqueue_style( 'jcarousel-styles', get_bloginfo('wpurl') . '/assets/jcarousel/skins/ie7/skin.css' );
add_action( 'print_footer_scripts', 'my_footer_script' );
}
}
// Add jQuery to footer
function my_footer_script() { ?>
<!-- only on front page -->
<?php if(is_front_page()) { ?>
<script type="text/javascript">
jQuery(function($) {
$('#carousel').jcarousel({
vertical: false,
scroll: 1,
wrap: "circular",
visible: 1,
auto: 5
});
});
</script>
<?php }
}
Example 3:
// =========================================
// = My Changes Below =
// =========================================
// load javascript and styles in footer or header
// only on http://websitesetuppro.com/how-to-use-classic-accordion-in-wordpress/
add_action('wp_enqueue_scripts', 'classicAccordion_code');
function classicAccordion_code() {
if(is_single( '538' )) {
wp_enqueue_script( 'classicAccordion', get_bloginfo('wpurl') . '/assets/grid-accordion/js/jquery.classicAccordion.fluid.js', array('jquery'), '1.0', true );
wp_enqueue_style( 'classic-accordion', get_bloginfo('wpurl') . '/assets/grid-accordion/css/classic-accordion.css' );
wp_enqueue_style( 'accordion_fluid', get_bloginfo('wpurl') . '/assets/grid-accordion/css/style.css' );
}
}
// only on http://websitesetuppro.com/full-screen-background-slideshow-in-wordpress/
add_action('wp_enqueue_scripts', 'my_jquery_animate_enhanced');
function my_jquery_animate_enhanced() {
if(is_single( '513' )) {
wp_deregister_script('jquery_animate_enhanced');
}
}
// on all pages of the site
add_action('wp_enqueue_scripts', 'add_my_code');
function add_my_code() {
add_action( 'print_footer_scripts', 'my_footer_script' );
}
// Add jQuery to footer
function my_footer_script() { ?>
<!-- on all pages of the site -->
<script type="text/javascript">
jQuery(function($) {
$('.gform_wrapper').find(':input').each(function() {
$(this).data('saveplaceholder', $(this).attr('placeholder'));
$(this).focusin(function() {
$(this).attr('placeholder', '');
});
$(this).focusout(function() {
$(this).attr('placeholder', $(this).data('saveplaceholder'));
});
});
});
</script>
<!-- only on http://websitesetuppro.com/how-to-use-classic-accordion-in-wordpress/ -->
<?php if(is_single( '538' )) { ?>
<script type="text/javascript">
var accordion;
jQuery(function($) {
$('.grid-accordion').classicAccordion({width:'100%', height:'100%', slideshow:true});
});
</script>
<?php }
}
Excerpts
To replace the ellipsis with Read more link
function new_excerpt_more($more) {
global $post;
return '<div class="more-link"><a href="'. get_permalink($post->ID) . '">Read More →</a></div>';
}
add_filter('excerpt_more', 'new_excerpt_more');
To have Read more link appear below every excerpt (kinda hard coding)
<p><a href="<?php the_permalink(); ?>" class="more-link">Read More →</a></p>
Clickable ellipsis
function clickable_ellipsis($content) {
$searchfor = '/\[(...)\]/';
$replacewith = '<a class="readmore" href="'.get_permalink().'">...more</a>';
$content = preg_replace($searchfor, $replacewith, $content, 1);
return $content;
}
add_filter('the_excerpt', 'clickable_ellipsis');
Expand images on hover
#it_widget_content-8 a img {
-webkit-transition: all 0.3s ease-in-out;
-moz-transition: all 0.3s ease-in-out;
-o-transition: all 0.3s ease-in-out;
-ms-transition: all 0.3s ease-in-out;
transition: all 0.3s ease-in-out;
}
#it_widget_content-8 a img:hover {
-webkit-transform: scale(1.1);
-moz-transform: scale(1.1);
-o-transform: scale(1.1);
transform: scale(1.1);
}
#builder-module-51ddf84be6d01-outer-wrapper,
#builder-module-51ddf84be6d01,
#builder-module-51ddf84be6d01 .widget-wrapper {
overflow: visible !important;
}
Live Demo (below the slider) | Source.
To show Featured Image outside the loop
Place this in a widget to show featured image attached for that content type (Post or Page or CPT etc.):
<?php
global $post;
if (has_post_thumbnail( $post->ID ) ) {
echo get_the_post_thumbnail($post->ID);
}
?>
Sticky header
Note: I provided the following code in Genesis forum here. Can easily be applied in any theme.
// =========================================
// = Fixed Header =
// =========================================
add_action('wp_enqueue_scripts', 'add_my_code');
function add_my_code() {
add_action( 'print_footer_scripts', 'my_footer_script' );
}
function my_footer_script() { ?>
<script type="text/javascript">
jQuery(function($) {
var $filter = $('#header');
var $filterSpacer = $('<div />', {
"class": "filter-drop-spacer",
"height": $filter.outerHeight()
});
if ($filter.size())
{
$(window).scroll(function ()
{
if (!$filter.hasClass('fix') && $(window).scrollTop() > $filter.offset().top)
{
$filter.before($filterSpacer);
$filter.addClass("fix");
}
else if ($filter.hasClass('fix') && $(window).scrollTop() < $filterSpacer.offset().top)
{
$filter.removeClass("fix");
$filterSpacer.remove();
}
});
}
});
</script>
<?php }
and
/*********************************************
Fixed Header
*********************************************/
#header.fix {
position: fixed;
top: 0;
left: 0;
z-index: 1000;
width: 100%;
max-width: 100%;
/*padding-top: 0;
padding-bottom: 0;*/
overflow: visible;
background: rgba(255, 255, 255, 0.92);
}
.admin-bar #header.fix {
top: 28px;
}
Font Awesome
At the top of style.css:
@import url(http://netdna.bootstrapcdn.com/font-awesome/3.1.1/css/font-awesome.css);
HTML:
<i class="icon-heart"></i> Support Us
To show font awesome icons before widget titles:
/*replace the content value with the
corresponding value from the list in source link*/
#text-2 h4.widget-title:before {
content: "\f087";
font-family: FontAwesome;
font-style: normal;
font-weight: normal;
text-decoration: inherit;
/*--adjust as necessary--*/
color: #000;
font-size: 18px;
padding-right: 0.5em;
position: absolute;
top: 0.2em;
left: 0;
}
#text-2 h4.widget-title {
padding-left: 1em;
}
For showing excerpts with thumbnails on Posts page
In index.php:
<?php if (has_post_thumbnail()) { ?>
<div class="front-page-thumbnail">
<a href="<?php the_permalink() ?>" rel="bookmark" title="<?php the_title_attribute(); ?>"><?php the_post_thumbnail('front-page-thumbnail'); ?></a>
</div>
<?php } ?>
<div class="post-content">
<?php the_excerpt(); ?>
</div>
In <span class="file-name">functions.php</span>:
add_image_size('front-page-thumbnail', 150, 150, true);
In style.css:
.front-page-thumbnail {
float: left;
margin-right: 1em;
margin-top: 1.7em;
}
.blog .entry-content {
clear: none;
margin-top: 0;
}
Full screen background
body {
background: url("images/bg-inner.jpg") no-repeat center center fixed #7F7F7F;
-webkit-background-size: cover;
-moz-background-size: cover;
-o-background-size: cover;
background-size: cover;
}
Get the first image in post automatically if featured image is not present
After installing and activating Get the Image plugin, in Left teaser image extension's functions.php, replace
<?php edit_post_link( '<img width="150" height="200" src="' . $this->_base_url . '/images/no-feature-image.jpg" class="alignleft teaser-thumb no-thumb" />', '<div class="teaser-thumb-wrapper">', '</div>' ) ; ?>
with
<?php if ( function_exists( 'get_the_image' ) ) { get_the_image( array( 'image_scan' => true, 'image_class' => 'alignleft', 'size' => 'it-teaser-thumb', 'default_image' => 'http://myranissen.dev/wp-content/uploads/2012/11/DSC_0040blog.jpg' ) ); } ?>
Styling for Gravity Forms form input fields
#gform_wrapper_3 input, #gform_wrapper_3 textarea {
background: #FFFFFF;
padding: 1em !important;
font-family: Arial, "Helvetica Neue", Helvetica, sans-serif;
}
.ginput_container .medium {
-webkit-box-shadow: 2px 2px 2px 0 #DDDDDD inset;
-moz-box-shadow: 2px 2px 2px 0 #DDDDDD inset;
box-shadow: 2px 2px 2px 0 #DDDDDD inset;
}
#input_2_1, #input_2_2, #input_2_3, #input_2_5 {
-webkit-box-shadow: 2px 2px 2px 0 #DDDDDD inset;
-moz-box-shadow: 2px 2px 2px 0 #DDDDDD inset;
box-shadow: 2px 2px 2px 0 #DDDDDD inset;
}
Hover effect (opacity change) for images
// =========================================
// = My Changes Below =
// =========================================
add_action('wp_enqueue_scripts', 'add_my_code');
function add_my_code() {
add_action( 'print_footer_scripts', 'my_footer_script' );
}
// Add jQuery to footer
function my_footer_script() { ?>
<!-- only on Posts page, archives and search listing pages -->
<?php if(is_home() || is_archive() || is_search()) { ?>
<script type="text/javascript">
jQuery(function($) {
$("img.listing-thumb").hover(
function () {
$(this).stop().fadeTo("fast", 0.7);
},
function () {
$(this).stop().fadeTo("fast", 1);
}
);
});
</script>
<?php }
}
If else
This code placed in a PHP enabled HTML module produces the same output as Header module:
<?php if (is_front_page()) : ?>
<h1 class="site-title"><a href="<?php bloginfo('url'); ?>"><?php bloginfo('name'); ?></a></h1>
<h3 class="site-tagline"><a href="<?php bloginfo('url'); ?>"><?php bloginfo('description'); ?></a></h5>
<?php else : ?>
<h3 class="site-title"><a href="<?php bloginfo('url'); ?>"><?php bloginfo('name'); ?></a></h1>
<h5 class="site-tagline"><a href="<?php bloginfo('url'); ?>"><?php bloginfo('description'); ?></a></h5>
<?php endif; ?>
Shadows
Drop shadow around container
.builder-container {
-moz-box-shadow: 0 0 25px #999;
-webkit-box-shadow: 0 0 25px #999;
-ms-filter: "progid:DXImageTransform.Microsoft.Glow(color=#999999,strength=3)";
filter:
progid:DXImageTransform.Microsoft.Shadow(color=#999999,direction=0,strength=3)
progid:DXImageTransform.Microsoft.Shadow(color=#999999,direction=90,strength=3)
progid:DXImageTransform.Microsoft.Shadow(color=#999999,direction=180,strength=3)
progid:DXImageTransform.Microsoft.Shadow(color=#999999,direction=270,strength=3);
box-shadow: 0 0 25px #999;
}
Subtle shadow
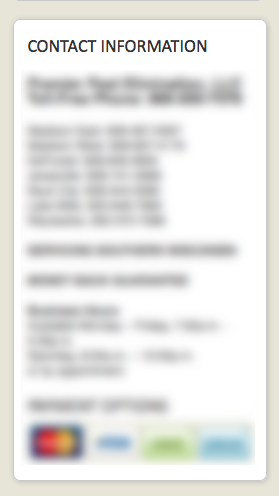
-webkit-box-shadow: 1px 1px 5px rgba(50, 50, 50, 0.23);
-moz-box-shadow: 1px 1px 5px rgba(50, 50, 50, 0.23);
box-shadow: 1px 1px 5px rgba(50, 50, 50, 0.23);
Shadow below an image
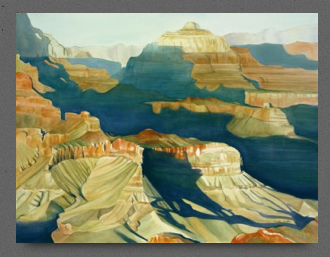
-webkit-box-shadow: 0 10px 10px -10px #000000;
-moz-box-shadow: 0 10px 10px -10px #000000;
box-shadow: 0 10px 10px -10px #000000;
Left and bottom shadow for post block
.hentry {
    background: #FFFFFF;
    padding: 1em;
    -webkit-box-shadow: -3px 3px 5px rgba(50, 50, 50, 0.8);
    -moz-box-shadow: -3px 3px 5px rgba(50, 50, 50, 0.8);
    box-shadow: -3px 3px 5px rgba(50, 50, 50, 0.8);
}
Shadow around content module
.builder-module-content-outer-wrapper {
    background: #fffffe; -moz-box-shadow: 0px 5px 30px #888; -webkit-box-shadow: 0px 5px 30px #888; box-shadow: 0px 5px 30px #888;
}
Polaroid effect for images in content module
.hentry img {
background: #FFFFFF;
padding: 5px;
-webkit-box-shadow: 0 10px 10px -10px #888888;
-moz-box-shadow: 0 10px 10px -10px #888888;
box-shadow: 0 10px 10px -10px #888888;
}
Thin polaroid around images in content module
Screenshot: (Observe any image in the content area of this site)
.hentry img {
background: #FFFFFF;
padding: 5px;
-webkit-box-shadow: rgba(0, 0, 0, 0.246094) 0px 1px 2px 0px, rgba(0, 0, 0, 0) 0px 0px 0px 0px, rgba(0, 0, 0, 0) 0px 0px 0px 0px;
box-shadow: rgba(0, 0, 0, 0.246094) 0px 1px 2px 0px, rgba(0, 0, 0, 0) 0px 0px 0px 0px, rgba(0, 0, 0, 0) 0px 0px 0px 0px;
border: 1px solid #ececec;
}
CSS Comments
/*********************************************
Comment
*********************************************/
PHP Comments
// =========================================
// = Builder Child Alternate Module Styles =
// =========================================
Opacity
#opacity-item {
-khtml-opacity: 0.5;
-moz-opacity: 0.5;
opacity: 0.5;
-ms-filter:"progid:DXImageTransform.Microsoft.Alpha(opacity=50)"; /* IE 8 */
filter: alpha(opacity=50); /* IE 4, 5, 6 and 7 */
zoom:1 /* so the element "hasLayout" or, to trigger "hasLayout" set a width or height */
}
To show page/post title outside loop
<?php echo get_the_title(); ?>
or,
<?php wp_title(''); ?>
Previous and Next post links
<div class="loop-footer">
<!-- Previous/Next page navigation -->
<div class="loop-utility clearfix">
<div class="alignleft"><?php next_post_link( '%link', '← Next Article' ); ?></div>
<div class="alignright"><?php previous_post_link( '%link', 'Previous Article →' ); ?></div>
</div>
</div>
Responsive background image CSS
background-image:url('../images/bg.png');
background-repeat:no-repeat;
background-size:contain;
background-position:center;
Rounded corners
-webkit-border-radius: 10px;
-moz-border-radius: 10px;
border-radius: 10px;
Separator using CSS
HTML:
<div class="seperator"></div>
CSS:
div.seperator{padding-top:15px;margin-bottom:0px;border-top:1px solid #EEE;clear:both;padding-bottom:0px;border-bottom:0;background:-moz-radial-gradient(50% 0, ellipse, rgba(0,0,0,0.2) 0%, rgba(255,255,255,0) 75%);background:-webkit-radial-gradient(50% 0, ellipse, rgba(0, 0, 0, 0.2) 0%, rgba(255, 255, 255, 0) 75%);background:-o-radial-gradient(50% 0, ellipse, rgba(0,0,0,0.2) 0%,rgba(255,255,255,0) 75%);background:-ms-radial-gradient(50% 0, ellipse, rgba(0,0,0,0.2) 0%,rgba(255,255,255,0) 75%);background:radial-gradient(50% 0, ellipse, rgba(0,0,0,0.2) 0%,rgba(255,255,255,0) 75%);}
Add new custom post thumbnail sizes
add_image_size('index-categories', 150, 150, true);
Text shadow
text-shadow: 1px 1px #FFFFFF;
To show current year (generally used in footer)
<?php echo date('Y'); ?>
Thanks for the code snippets…and perfect timing since the WebDesign’s WordPress Developers Course just ended! I’ll put them to immediate use 🙂
Carol
I’m using iThemes Builder Air Child-theme with a self designed custom png image as the header, I want to make it clickable – to lead back to the home page. I’m wondering how to do this?
I’m also wondering whether use of an image as a header instead of the default header widget makes my site less SEO friendly.
Grateful for your thoughts.
Thanks Sridhar these are great
Super helpful thank you very much Sridhar 🙂
You have the “Previous and Next post links” in your Listing.
Do you have got a code for “Previous and Next images links” or “Previous and Next attachments links”?
Thank you a lot!
wow, I am in heaven! Not sure where to ask but do you have any advice for setting up a lightweight facebook open graph function to help push images to the thumbnail option in facebook post or page links, we always get the wrong images popping up and we don’t want to hard code just a logo.
Try WordPress SEO by Yoast.
I am using that right now, but home page links always picks up the slider no matter what I put in the backend settings in Yoast. I have tried various plugins and have had no success. If I hard code in header.php the 200×200 image which Yoast suggests to upload in wordpress as a default image, I am thinking it might supercede all images and be the only thing that posts in facebook links. Got stuck there.
oh well, I am afraid I can’t help you on this as I’ve stopped using Builder. Have you tried iThemes forum? What does Ronald say?